#HTML
#CSS
#JavaScript
قم فقط بتبديل CLIENT_ID إلى معرف العميل الخاص بحساب PayPal
HTML
<div id="locked-card" class="card-container">
<div class="card-header">
<i class="fas fa-lock card-lock"></i>
<h2>This card is locked</h2>
</div>
<div class="card-body">
<p>To unlock, please pay $10 via PayPal:</p>
<div id="paypal-button-container"></div>
</div>
</div>
<div id="unlocked-card" class="card-container" style="display: none;">
<div class="card-header">
<h2>This card is now unlocked!</h2>
</div>
<div class="card-body">
<p>Thank you for your payment.</p>
<a href="other-page.html" class="card-button">Go to Other Page</a>
</div>
</div>
<script src="https://www.paypal.com/sdk/js?client-id=CLIENT_ID"></script>
<style>
.card-container {
width: 400px;
border: 1px solid #ccc;
border-radius: 5px;
margin: 20px auto;
padding: 20px;
}
.card-header {
display: flex;
align-items: center;
}
.card-lock {
font-size: 24px;
margin-right: 10px;
}
.card-body {
margin-top: 20px;
}
.card-button {
padding: 10px 20px;
background-color: #0099ff;
color: #fff;
text-decoration: none;
border-radius: 5px;
display: inline-block;
margin-top: 20px;
}
.card-button:hover {
background-color: #0077cc;
}
</style>
<script>
paypal.Buttons({
// Set up the transaction
createOrder: function(data, actions) {
return actions.order.create({
purchase_units: [{
amount: {
currency_code: 'USD',
value: '10.00'
}
}]
});
},
onApprove: function(data, actions) {
return actions.order.capture().then(function(details) {
if (details.purchase_units[0].amount.value === '10.00') {
document.getElementById('locked-card').style.display = 'none';
document.getElementById('unlocked-card').style.display = 'block';
localStorage.setItem('payment_verified', 'true');
} else {
alert('Incorrect payment amount. Please pay $10.');
}
});
},
onError: function(err) {
alert('Payment failed!');
}
}).render('#paypal-button-container');
window.onload = function() {
checkPaymentStatus();
}
function checkPaymentStatus() {
var paymentVerified = localStorage.getItem('payment_verified');
if (paymentVerified === 'true') {
document.getElementById('locked-card').style.display = 'none';
document.getElementById('unlocked-card').style.display = 'block';
} else {
document.getElementById('paypal-button').disabled = true;
}
}
</script>
This card is locked
To unlock, please pay $10 via PayPal:
#HTML
#CSS
#JavaScript
لعبة memory game بسيطة كود اللعبة جاهز للإستخدام في أي مشروع تريده يمكنك التغيير من الألوان إلى الصور على حسب رغبتك أتمنى أن تعجبكم اللعبة
HTML
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title></title>
<script src="https://cdn.jsdelivr.net/npm/@tensorflow/tfjs@3.11.0/dist/tf.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/@tensorflow-models/mobilenet@2.0.4/dist/mobilenet.min.js"></script>
</head>
<body>
<h1>تحليل الصور باستخدام الذكاء الاصطناعي</h1>
<input type="file" id="image-selector" onchange="loadImage()" />
<img id="selected-image" src="" />
<button onclick="classifyImage()">تصنيف الصورة</button>
<script>
let model;
async function loadModel() {
model = await mobilenet.load();
}
loadModel();
function loadImage() {
const reader = new FileReader();
reader.onload = function () {
const dataURL = reader.result;
const image = document.getElementById('selected-image');
image.src = dataURL;
image.style.display = 'block';
}
const file = document.getElementById('image-selector').files[0];
reader.readAsDataURL(file);
}
async function classifyImage() {
const image = document.getElementById('selected-image');
const result = await model.classify(image);
console.log(result);
alert(`هذه الصورة تحتوي على ${result[0].className}`);
}
</script>
</body>
</html>
تحليل الصور باستخدام الذكاء الاصطناعي
#HTML
#CSS
#JavaScript
لعبة memory game بسيطة كود اللعبة جاهز للإستخدام في أي مشروع تريده يمكنك التغيير من الألوان إلى الصور على حسب رغبتك أتمنى أن تعجبكم اللعبة
HTML
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Memory Game</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f2f2f2;
margin: 0;
padding: 0;
}
h1 {
text-align: center;
}
#game-container {
display: flex;
flex-direction: column;
align-items: center;
height: 500px;
}
#card-container {
display: flex;
flex-wrap: wrap;
justify-content: center;
align-items: center;
width: 400px;
height: 400px;
margin: 20px;
padding: 10px;
border: 5px solid #3498db;
}
.card {
width: calc(25% - 10px);
height: calc(25% - 10px);
background-color: #e74c3c;
margin: 5px;
border-radius: 5px;
cursor: pointer;
transition: all 0.2s ease-in-out;
}
.card:hover {
background-color: #c0392b;
}
.card.matched {
background-color: #2ecc71;
pointer-events: none;
}
#button-container {
display: flex;
justify-content: center;
align-items: center;
width: 100%;
margin: 10px;
}
button {
background-color: #3498db;
color: #fff;
border: none;
border-radius: 5px;
padding: 10px;
margin: 10px;
cursor: pointer;
transition: all 0.2s ease-in-out;
}
button:hover {
background-color: #2980b9;
}
#start-button:hover {
transform: scale(1.1);
}
#reset-button:hover {
transform: scale(1.1);
}
#message-container {
font-size: 24px;
font-weight: bold;
text-align: center;
color: #3498db;
}
@media (max-width: 768px) {
#card-container {
width: 300px;
height: 300px;
}
.card {
width: calc(33.33% - 10px);
height: calc(33.33% - 10px);
}
}
</style>
</head>
<body>
<h1>Memory Game</h1>
<div id="game-container">
<div id="card-container"></div>
<div id="button-container">
<button id="start-button">Start</button>
<button id="reset-button">Reset</button>
</div>
<div id="message-container"></div>
</div>
<script>
const cardContainer = document.getElementById("card-container");
const startButton = document.getElementById("start-button");
const resetButton = document.getElementById("reset-button");
const messageContainer = document.getElementById("message-container");
const colors = [
"red",
"green",
"blue",
"yellow",
"orange",
"purple",
"pink",
"brown"
];
let cards = [];
let flippedCards = [];
let matchedCards = [];
let canFlip = false;
let numMatches = 0;
function createCards() {
for (let i = 0; i < colors.length; i++) {
const cardOne = createCard(colors[i]);
const cardTwo = createCard(colors[i]);
cards.push(cardOne);
cards.push(cardTwo);
}
shuffleCards();
}
function createCard(color) {
const card = document.createElement("div");
card.classList.add("card");
card.dataset.color = color;
card.addEventListener("click", () => {
if (canFlip && !card.classList.contains("matched") && !flippedCards.includes(card)) {
flipCard(card);
flippedCards.push(card);
if (flippedCards.length === 2) {
canFlip = false;
checkMatch();
}
}
});
return card;
}
function shuffleCards() {
for (let i = cards.length - 1; i > 0; i--) {
const j = Math.floor(Math.random() * (i + 1));
[cards[i], cards[j]] = [cards[j], cards[i]];
}
}
function flipCard(card) {
card.style.backgroundColor = card.dataset.color;
}
function checkMatch() {
if (flippedCards[0].dataset.color === flippedCards[1].dataset.color) {
flippedCards[0].classList.add("matched");
flippedCards[1].classList.add("matched");
matchedCards.push(flippedCards[0]);
matchedCards.push(flippedCards[1]);
numMatches++;
if (numMatches === colors.length) {
endGame();
} else {
canFlip = true;
flippedCards = [];
}
} else {
setTimeout(() => {
flippedCards.forEach(card => {
card.style.backgroundColor = "#e74c3c";
});
flippedCards = [];
canFlip = true;
}, 1000);
}
}
function startGame() {
createCards();
cardContainer.innerHTML = "";
cards.forEach(card => {
cardContainer.appendChild(card);
});
startButton.disabled = true;
resetButton.disabled = false;
messageContainer.innerHTML = "";
canFlip = true;
}
function resetGame() {
cards = [];
flippedCards = [];
matchedCards = [];
numMatches = 0;
cardContainer.innerHTML = "";
startButton.disabled = false;
resetButton.disabled = true;
messageContainer.innerHTML = "";
}
function endGame() {
messageContainer.innerHTML = "Congratulations! You won!";
}
startButton.addEventListener("click", startGame);
resetButton.addEventListener("click", resetGame);
</script>
</body>
</html>
Memory Game
#CSS
كود لرسم وجه غاضب مع animation
الدخان الذي يبين بأنه غاضب جدا فقط بلغة html
و css
أتمنى أن تعجبكم الفكرة وتدعمونا فقط إضغظ على run لرأية نتيجة الكود وإذا أعجبك يمكنك نسخه والتعلم منه والتعديل عليه بطريقة أفضل
HTML
<div class="smoking"> <span style="--var:3"></span> <span style="--var:5"></span> <span style="--var:1"></span> <span style="--var:8"></span> <span style="--var:13"></span> <span style="--var:2"></span> <span style="--var:7"></span> <span style="--var:9"></span> <span style="--var:6"></span> <span style="--var:4"></span> <span style="--var:12"></span> <span style="--var:18"></span> <span style="--var:10"></span> <span style="--var:14"></span> <span style="--var:11"></span> <span style="--var:17"></span> <span style="--var:15"></span> <span style="--var:16"></span> </div> <div class="face"> <div class="mouth"></div> <div class="brow1"></div> <div class="brow2"></div> </div>
CSS
.smoking { position: relative; z-index: 1; padding: 0 20px; } .smoking span { position: absolute; bottom: -125px; left: 97px; display: block; margin: 0 2px 50px; min-width: 8px; height: 120px; background: #000; border-radius: 50%; animation: smoking 5s linear infinite; opacity: 0; filter: blur(8px); animation-delay: calc(var(--var) * -0.5s); } @keyframes smoking { 0% { transform: translateY(0) scaleX(1); opacity: 0; } 15% { opacity: 1; } 50% { transform: translateY(-150px) scaleX(5); } 95% { opacity: 0; } 100% { transform: translateY(-300px) scaleX(10); } } .face { width: 200px; height: 200px; background: radial-gradient(red, rgb(255, 255, 8)); border-radius: 50%; position: relative; } .face::before { content: ""; width: 20px; height: 20px; background-color: #000; border-radius: 50%; position: absolute; left: 42px; top: 78px; transform: rotate(20deg); } .face::after { content: ""; width: 20px; height: 20px; background-color: #000; border-radius: 50%; position: absolute; left: 129px; top: 78px; transform: rotate(20deg); } .mouth { width: 100px; height: 100px; background-color: transparent; border-top: 10px solid #000; border-radius: 51px; position: relative; top: 124px; left: 49px; } .brow1 { width: 28px; height: 10px; background-color: #000; position: relative; border-radius: 10px; left: 117px; top: -48px; transform: rotate(164deg); } .brow2 { width: 28px; height: 10px; background-color: #000; position: relative; border-radius: 10px; left: 46px; top: -57px; transform: rotate(196deg); }
#SVG
تعرف على كيفية عمل تموج فقط باستخدام SVG
بدون تدخل CSS
HTML
<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 1440 280"> <path fill-opacity="1"> <animate attributeName="d" dur="20000ms" repeatCount="indefinite" values= " M0,160L48,181.3C96,203,192,245,288,261.3C384,277,480,267,576,234.7C672,203,768,149,864,117.3C960,85,1056,75,1152,90.7C1248,107,1344,149,1392,170.7L1440,192L1440,320L1392,320C1344,320,1248,320,1152, 320C1056,320,960,320,864,320C768,320,672,320,576,320C480,320,384,320,288,320C192,320,96,320,48,320L0, 320Z; M0,160L48,181.3C96,203,192,245,288,234.7C384,224,480,160,576,133.3C672,107,768,117,864,138.7C960,160,1056,192,1152,197.3C1248,203,1344,181,1392,170.7L1440,160L1440,320L1392,320C1344,320,1248,320,1152, 320C1056,320,960,320,864,320C768,320,672,320,576,320C480,320,384,320,288,320C192,320,96,320,48, 320L0,320Z;M0,64L48,74.7C96,85,192,107,288,133.3C384,160,480,192,576,170.7C672,149,768,75,864,80C960, 85,1056,171,1152,181.3C1248,192,1344,128,1392,96L1440,64L1440,320L1392,320C1344,320,1248,320,1152,320C1056,320, 960,320,864,320C768,320,672,320,576,320C480,320,384,320,288,320C192,320,96,320,48,320L0,320Z; M0,160L48,181.3C96,203,192,245,288,261.3C384,277,480,267,576,234.7C672,203,768,149,864,117.3C960,85,1056, 75,1152,90.7C1248,107,1344,149,1392,170.7L1440,192L1440,320L1392,320C1344,320,1248,320,1152,320C1056,320,960, 320,864,320C768,320,672,320,576,320C480,320,384,320,288,320C192,320,96,320,48,320L0,320Z;"> </animate> </path> </svg>
#JavaScript
هل تعرف يمكنك الكتابة هكذا في لغة JavaScript
const arr = [1, 2, 3, 4, 5] let i = arr.length for (;i --> 0;) console.log(arr[i])
5
4
3
2
1
#CSS
كود HTML AND CSS
لزر الإشعارات
HTML
<button class="badg">Email</button>
CSS
.badg { background-color: #36e; color: #fff; width: 100px; height: 45px; border: none; outline: none; font-weight: bold; border-radius: 5px; position: relative; } .badg::before { content: "5"; position: absolute; width: 25px; height: 25px; background-color: red; border-radius: 50%; top: -21%; left: 83%; }
#CSS
كود Animation
مثل الكرة.
HTML
<div class="ball"></div>
CSS
.ball { background-color: #fd5c5c; width: 50px; height: 50px; border-radius: 50%; animation: ball .5s infinite alternate; } @keyframes ball { from { transform: translateY(0); } from { transform: translateY(20px); } }
#CSS
كود Animation
دقات القلب تنويه إستعملت أيقونات Bootstrap
يمكنك إستعمال أيقونات مكتبة أخرى أو تستعمل صورة فقط.
HTML
<div class="bi bi-heart-fill" id="an_heart"></div>
CSS
#an_heart { color: #fd5c5c; font-size: 50px; animation: heart 1s infinite; display: inline-block; } @keyframes heart { from { transform: scale(0); } to { transform: scale(1); } }
#CSS
كود Animation
عبارة عن Loading رائع.
HTML
<div class="spinner"></div>
CSS
.spinner { width: 70px; height: 70px; border-width: 5px; border-color: #fd5c5c #ccc #fd5c5c #ccc; border-style: solid; border-radius: 50%; animation: spinner 1s infinite; } @keyframes spinner { from { transform: rotate(0); } to { transform: rotate(360deg); } }
#JavaScript
كود لغة JavaScript
يقوم بنسخ النص الموجود
داخل حقل الإدخال قم بإدراج نص داخل حقل الإدخال و إضغظ على الزر copy
سوف ينسخ النص الذي أدخلته.
HTML
<input type="text" id="txt" placeholder="Enter your text..."> <button id="copy">copy</button>
JavaScript
let copy = document.getElementById("copy"); let inp = document.getElementById("txt") copy.addEventListener("click", function () { inp.select(); document.execCommand("copy", false) })
#CSS
رسم شكل عبارة عن جوهرة فقط بإستخدام CSS
HTML
<div class="dm"></div>
CSS
.dm { border-style: solid; border-color: transparent transparent #34e transparent; border-width: 0 25px 25px 25px; height: 0; width: 50px; box-sizing: content-box; position: relative; margin: 20px 0 50px 0; } .dm::before { content: ""; position: absolute; top: 25px; left: -25px; width: 0; height: 0; border-style: solid; border-color: #34e transparent transparent transparent; border-width: 70px 50px 0 50px; }
#CSS
رسم شكل عبارة عن قلب فقط بإستخدام CSS
HTML
<div class="dm"></div>
CSS
.hr { height: 90px; width: 100px; position: relative; } .hr::before, .hr::after { content: ""; position: absolute; top: 0; left: 50px; width: 50px; height: 80px; background: #f45; border-radius: 50px 50px 0 0; transform: rotate(-45deg); transform-origin: 0 100%; } .hr::after { left: 0; transform: rotate(45deg); transform-origin: 100% 100%; }
#SVG
رسم شكل بسيط جدا مثل الوجه بإستخدام SVG
HTML
<svg width="100" height="100"> <rect x="10" y="20" fill="red" ry="10" rx="10" width="100" height="100"/> <circle cy="50" cx="50" r="10" fill="#000"/> <circle cy="50" cx="50" r="5" fill="#fff"/> <circle cy="50" cx="80" r="10" fill="black"/> <circle cy="50" cx="80" r="5" fill="#fff"/> <line stroke="#000" stroke-width="10" y1="30" y2="83" x1="8000" x2="21"> </svg>
#CSS
إضافة تأتير تأتير تدرج الألوان على النص.
HTML
<h1 class="title">Ayoub Ayoau</h1>
CSS
.title { background: linear-gradient(0deg, #f45, #34e); background-clip: text; -webkit-background-clip: text; -webkit-text-fill-color: transparent; }
Ayoub Ayoau
#HTML
تعرف على عنصر map
الذي يمكنك من تقسيم صورة واحدة إلى عدة روابط.
HTML
<img width="100px" src="./assets/img/ayoub.jpg" usemap="#demo" alt=""> <map name="demo"> <area shape="circle" coords="12, 23, 34, 5" href="https://google.com" alt=""> <area shape="circle" coords="68, 54, 93, 7" href="https://facebook.com" alt=""> <area shape="circle" coords="52, 53, 34, 3" href="https://instagrma.com" alt=""> </map>
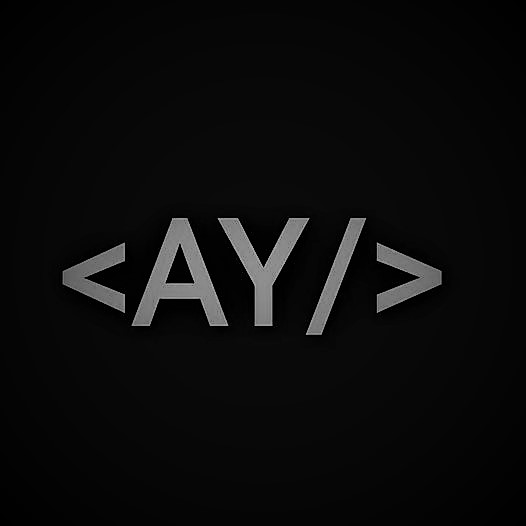
#HTML
#CSS
#JavaScript
إنشاء dropDown بسيطة فقط بإستخدام HTML CSS JavaScript
HTML
<div class="dropdown"> <button onclick="dropDown()" class="dropbtn">Dropdown</button> <div id="myDropdown" class="dropdown-content"> <a href="#">Link 1</a> <a href="#">Link 2</a> <a href="#">Link 3</a> </div> </div>
CSS
.dropbtn { background-color: #f45; color: white; padding: 8px; font-size: 16px; border: none; cursor: pointer; border-radius: 5px; font-weight: bold; } .dropbtn:hover, .dropbtn:focus { background-color: #ec4c4c; } .dropdown { position: relative; display: inline-block; } .dropdown-content { display: none; position: absolute; background-color: #ffff; min-width: 160px; box-shadow: 0px 8px 16px 0px rgba(0,0,0,0.2); z-index: 1; } .dropdown-content a { color: black; padding: 12px 16px; text-decoration: none; display: block; } .dropdown-content a:hover { background-color: #ddd } .show { display:block; }
JavaScript
function dropDown() { document.getElementById("myDropdown").classList.toggle("show"); } window.onclick = function(event) { if (!event.target.matches('.dropbtn')) { var dropdowns = document.getElementsByClassName("dropdown-content"); var i; for (i = 0; i < dropdowns.length; i++) { var openDropdown = dropdowns[i]; if (openDropdown.classList.contains('show')) { openDropdown.classList.remove('show'); } } } }
#CSS
إنشاء شكل menu icon بإستخدام CSS
HTML
<div class="line1"></div> <div class="line2"></div> <div class="line3"></div>
CSS
.line1 { width: 30px; height: 3px; background-color: black; margin: 6px 0; } .line2 { width: 25px; height: 3px; background-color: black; margin: 6px 0; } .line3 { width: 30px; height: 3px; background-color: black; margin: 6px 0; }
#CSS
عمل شكل بطارية مع كرحة الشحن فقط بلغة ال CSS
HTML
<div class="battery"></div>
CSS
.battery { border: 5px solid #ccc; border-radius: 10px; height: 70px; position: relative; width: 200px; } .battery::after { content: ""; background-color: #ccc; border-radius: 5px; height: 30px; width: 15px; position: absolute; right: -23px; top: 30%; transform: translate(-50%); } .battery::before { content: ""; animation: fill 3s linear infinite; background-color: #0ed02e; border-radius: 5px; height: 52px; width: 40%; position: absolute; left: 4px; top: 4px; } @keyframes fill { 0% { width: 50%; } 50% { width: 70%; } 100% { width: 90%; } }
جميع العناصر
- All 14
- HTML 1
- CSS 9
- JavaScript 2
- Python 0
- jQuery 0
- HTML CSS JS 1
- SVG 1